Yeah… I had to make that pun!
Ok so we are still spinning around the subject of loops in Powershell. This time as you might suspect we’re going to take a look at For and While.
Now I don’t typically use these but figured we’d talk a bit about them so that I have a legitimate reason for that pun 😉
For loop
for ($i = 0; $i -lt 4; $i++){
Start-Process Notepad
$i
}
Above is a simple For loop that will start Notepad. Can you guess how many Notepads will be opened?
The condition (the part between the parentheses) has three parts separated by semicolons.
In the first part we specify a variable and assign a number to it, in this case 0.
Next we decide for how long the loop should run, I choose to continue looping as long as $i is less than 4.
Last part is making sure that the value of $i changes so that the loop eventually stops. I used $i++, which means that $i will increase by 1 each cycle.
Besides starting Notepad a bunch of times we also return the value of $i, returned values from the example:
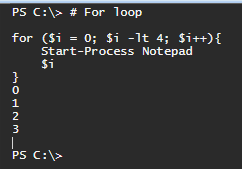
While loop
$i = 0
while($i -le 4){
Start-Process notepad
$i
$i++
}
In this While loop example we are again using $i with an initial value of 0, but it has to be specified outside of the While statement.
You can read the code simply as “while (this is true) {do this}”.
Instead of -lt (less than) we’re using -le (less than or equal to) and this gives us the output:
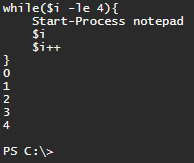
Do While
$i = 6
do{
Start-Process notepad
$i
$i++
}
while($i -le 5)
Do While loop is basically a normal While loop but with the difference that a Do While will always run its code block at least once, even if the While statement is false from the start like in the above example.
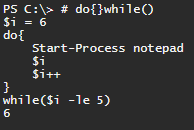
Bonus round – Do Until
$i = 1
do{
Start-Process notepad
$i
$i++
}
until($i -eq 5)
Very similar to the While loop but instead of doing something WHILE the statement is true, Do Until does something UNTIL the statement is true.
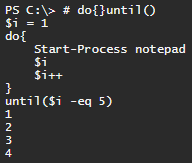
General information about loops
First of, loops are great, please take the effort to learn how to use them. It can save so much time and make daunting tasks quick and easy.
You can break out of loops early if you want by using the command Break. Useful for stopping a loop if the goal is achieved earlier than expected or if you encounter an error.
It is also possible to skip ahead to the next cycle without executing all or parts of the current cycle by using the command Continue.
$num = 1..5
foreach($n in $num){
"Start of loop $n :)"
if($n -eq 3){
Continue
}
elseif($n -eq 4){
"Woops I stopped early :S"
Break
}
"End of loop $n :("
}
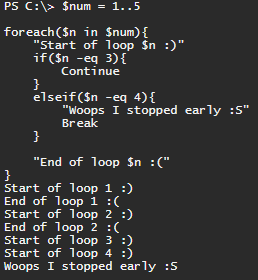
As mentioned in the last post about loops, you can manually break out of them by pressing CTRL + C.
If you are using Powershell 7 (not officially available in ISE), then you can use -Parallel on Foreach-Object to run the loop with several threads, allowing your loop to be even quicker!
If your stuck in ISE with PowerShell 5 you can still get multithreading in your loops but you have to do the work yourself, we might take a peak at it in a future post 🙂
There is so much more that can be said, showed and explained about loops in Powershell but I try to make these posts, especially the beginner tutorials, short and interesting. It should be easy and fun learning Powershell. We can geek about the deep questions and problems when you get there 😉
We’ll be seeing different loops, but mainly Foreach/Foreach-Object, in other posts since I use them a lot.
As always, continue to learn and evolve your skills, see you in the next one!
Do you want to know more? Here is a list of tutorials to check next